Multiple items
module List
from Microsoft.FSharp.Collections
--------------------
type List<'T> =
| ( [] )
| ( :: ) of Head: 'T * Tail: 'T list
interface IEnumerable
interface IEnumerable<'T>
member Head : 'T
member IsEmpty : bool
member Item : index:int -> 'T with get
member Length : int
member Tail : 'T list
static member Cons : head:'T * tail:'T list -> 'T list
static member Empty : 'T list
Full name: Microsoft.FSharp.Collections.List<_>
val sum : list:'T list -> 'T (requires member ( + ) and member get_Zero)
Full name: Microsoft.FSharp.Collections.List.sum
val printfn : format:Printf.TextWriterFormat<'T> -> 'T
Full name: Microsoft.FSharp.Core.ExtraTopLevelOperators.printfn
val square : x:int -> int
Full name: Document.square
val x : int
val sq : int
Full name: Document.sq
type Person =
{First: string;
Last: string;}
Full name: Document.Person
Person.First: string
Multiple items
val string : value:'T -> string
Full name: Microsoft.FSharp.Core.Operators.string
--------------------
type string = System.String
Full name: Microsoft.FSharp.Core.string
Person.Last: string
type Employee =
| Worker of Person
| Manager of Employee list
Full name: Document.Employee
union case Employee.Worker: Person -> Employee
union case Employee.Manager: Employee list -> Employee
type 'T list = List<'T>
Full name: Microsoft.FSharp.Collections.list<_>
val jdoe : Person
Full name: Document.jdoe
val worker : Employee
Full name: Document.worker
Multiple items
val int : value:'T -> int (requires member op_Explicit)
Full name: Microsoft.FSharp.Core.Operators.int
--------------------
type int = int32
Full name: Microsoft.FSharp.Core.int
--------------------
type int<'Measure> = int
Full name: Microsoft.FSharp.Core.int<_>
namespace System
MTUG Dublin
F# and Open Source
Andrea Magnorsky
Digital Furnace Games ␣ ▀ ␣ BatCat Games ␣ ▀ ␣ GameCraft Foundation
Working on OniKira: Demon Killer
Available on Steam Early Access
F# OSS and you
Latest announcements, Big wins !
- Great for language adoption
- Visual Studio community with extensions
- CLR in a package FTW
1:
2:
3:
4:
5:
6:
7:
8:
9:
10:
11:
12:
13:
14:
15:
16:
17:
18:
|
// one-liners
[1..100] |> List.sum |> printfn "sum=%d"
// no curly braces, semicolons or parentheses
let square x = x * x
let sq = square 42
// simple types in one line
type Person = {First:string; Last:string}
// complex types in a few lines
type Employee =
| Worker of Person
| Manager of Employee list
// type inference
let jdoe = {First="John";Last="Doe"}
let worker = Worker jdoe
|
Visit F# for Fun and Profit for more examples
Some examples
Light syntax, POCOs
C#
public class Person
{
public Person(string name, int age)
{
_name = name;
_age = age;
}
private readonly string _name;
private readonly int _age;
public string Name
{
get { return _name; }
}
public int Age
{
get { return _age; }
}
} |
F#
1:
2:
3:
4:
5:
6:
7:
|
type Person( name:string, age:int) =
/// Full name
member person.Name = name
/// Age in years
member person.Age = age
|
C#
using NUnit.Framework;
[TestFixture]
public class MathTest
{
[Test]
public void TwoPlusTwoShouldEqualFour()
{
Assert.AreEqual(2 + 2, 4);
}
} |
F#
1:
2:
3:
4:
5:
6:
|
module MathTest =
open NUnit.Framework
let [<Test>] ``2 + 2 should equal 4``() =
Assert.AreEqual(2 + 2, 4)
|
FsCheck
1:
2:
3:
4:
5:
6:
7:
|
module MathTest =
open FsCheck.Nunit
[<Property>]
let ``Given a, a + a should equal a * 2`` ( a: int) =
a + a = 2 * a
|
You can use FsCheck from F# and C#.
Some examples
C# <-> F# Interop
C# consuming F# code
Use namespaces in F# or prefix with global::YourModuleName
using System;
class Program
{
static void Main(string[] args)
{
var s = Calculator.Calc.add("4 4", "+");
Console.WriteLine("The sum is {0}", s);
}
} |
and the F# side
1:
2:
3:
4:
5:
6:
|
namespace Calculator
module Calc =
open System
let add numbers delimiter =
// Do stuff to add numbers
|
F# consuming C# code
1:
2:
3:
4:
5:
6:
|
module MathTest =
open NUnit.Framework
let [<Test>] ``2 + 2 should equal 4``() =
Assert.AreEqual(2 + 2, 4)
|
Like games? Dublin GameCraft on the 6th of December @ Microsoft
F# and games workshop from 10am to noon.
Functional Kats Monthly meetup
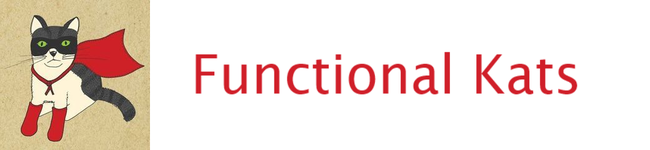